Get a jump start on your python career with this tutorial which covers the basics of python.
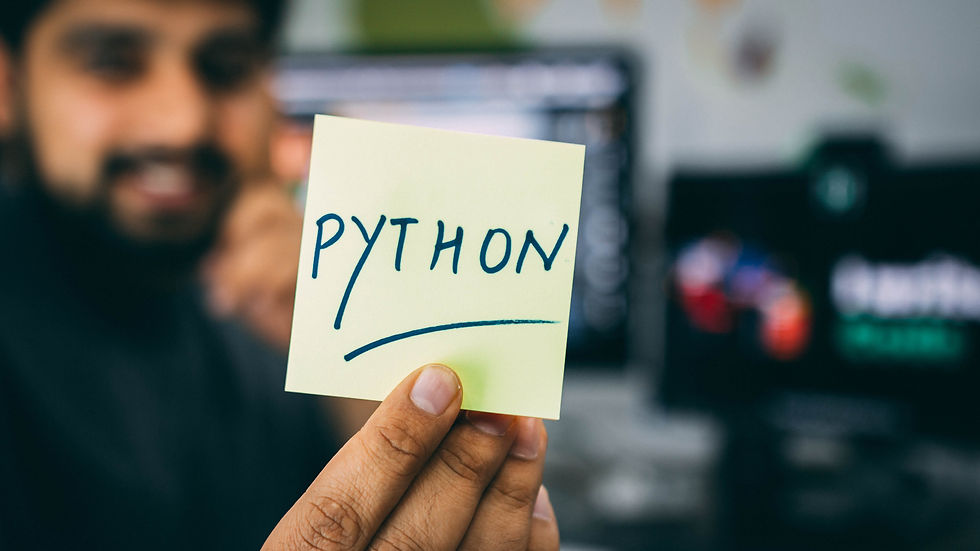
What is python?
Python is a high-level, object-oriented, interpreted programming language, which has garnered worldwide attention. Python is an open-source object-oriented programming language. It is the fastest-growing programming language. Python’s popularity surpassed that of C# in 2018 — just like it surpassed PHP in 2017.
Why learn Python?
Python’s syntax is very easy to understand. The lines of code required for a task is less compared to other languages. For example — If I have to print “Welcome To LinkedIn!” all you have to type is :
print("Welcome To LinkedIn")
Some more features of Python are:-
Simple and easy to learn
Free and Open Source
Portable
Supports different programming paradigm
Extensible
Let us now start coding in Python.
SYNTAX CONVENTIONALITY
It’s important to get familiar with the syntax of the codes in python before starting the coding process. Python is a case sensitive language. It makes use of whitespace for code structuring and marking logical breaks in the code (In contrast to other programming languages that use braces).
The end of the line marks the end of a statement, so it does not require a semicolon at end of each statement.
Whitespace at the beginning of the line is important. This is called indentation.
Leading whitespace (spaces and tabs) at the beginning of the logical line is used to determine the indentation level of the logical line.
Statements that go together must have the same indentation. Each such set of statements is called a block. Let’s understand indentation with the following example:
for x in alist:
if x < 100:
print(x)
else:
print(-x)
is similar to <--- Let us compare with other language
for x in alist
{
if x < 100
{
print(x)
}
else
{
print(-x)
}
}
print("Now we understand indentation")
How to add comments in Python
One line comments are denoted by # at the start of the line, multiple lines or a block comment start with ‘’’ and end with ‘’’.
Take a look at the following example :
# this is a single line comment
# print("I will not be printed, I am a single line comment")
'''
This is a block comment 3 single quotes
print("We are in a comment")
print ("We are still in a comment")
'''
print("We are out of the comment")
Variables in Python
Variables are nothing but reserved memory locations to store values. This means that when you create a variable you reserve some space in memory. In Python, you don’t need to declare variables before using it, unlike other languages like Java, C etc.
Assigning values to a variable
Python variables do not need an explicit declaration to reserve memory space. The declaration happens automatically when you assign a value to a variable. The equal sign (=) is used to assign values to variables.
S = 10
print(S)
This will assign the value ‘10’ to S and will print it. Try it yourself!
The next part is understanding Data Types in Python.
Data Types in Python :
Python supports various data types, these data types define the operations possible on the variables and the storage method. Built-in data types are Integer, Floating point, String, Boolean Values, Date and Time. Additional data structures are Tuples, Lists, Dictionary.
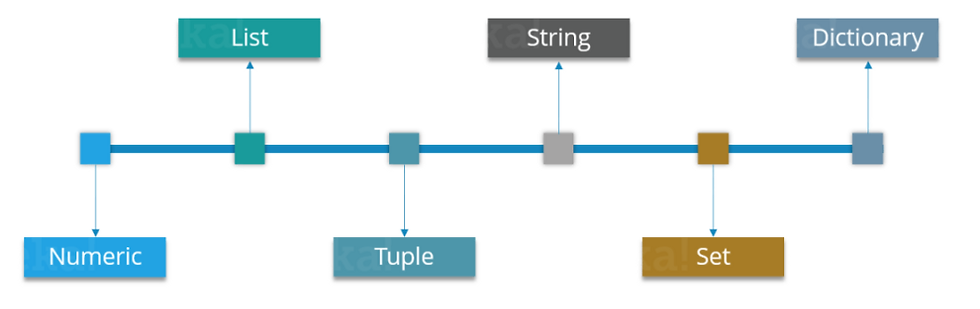
We’ll discuss each of these in detail. Let’s start with Numeric Data Type.
Numeric Data Types
Just as the name suggests Numeric Data Types store numeric values. They are immutable data types, this means that you cannot change their value. Python supports the following Numeric data types:-
Integer Type: It holds all the integer values i.e. all the positive and negative whole numbers, for example — 10.
X = 45
print(x)
Float Type: It holds the real numbers and are represented by a decimal and sometimes even scientific notations with E or e indicating the power of 10
y = 14.56
print(y)
Converting one Data Type to another: You can easily perform type conversion in Python. For example, you can convert the integer value to a float value and vice-versa. Consider the following examples:-
A = 10
#Convert into float data type
B = float(A)
print(B)
The code above will convert an integer value to a float type. Similarly, you can convert a float value to integer type:-
A = 10.56
#Covert into integer data type
B = int(A)
print(B)
Now let’s understand what lists are in Python.
Lists
Lists, along with dictionary, are perhaps the most important data types.
A list contains items separated by commas and enclosed within square brackets ( [ ] ).
All the items belonging to a list can be of different data type.
Lists are similar to arrays in the C language. You can consider the Lists as Arrays in C, but in List, you can store elements of different types, but in Array, all the elements should of the same type.
The plus ( + ) sign is the list concatenation operator, and the asterisk ( * ) is the repetition operator.
Consider the example below:
list_ = [ 'abcd', 786 , 2.23, 'ISB', 70.2 ]
tinylist = [123, 'ISB']
print (list_) # Prints complete list
print (list_[0]) # Prints first element of the list
print (list_[1:3]) # Prints elements starting from 2nd till 3rd
print (list_[2:]) # Prints elements starting from 3rd element
print (tinylist * 2) # Prints list two times
print (list_ + tinylist) # Prints concatenated lists
print (len(list_)) # Prints the length of the list
Notice that the list_ contains both words as well as numbers. Let us look at some more operations that can be performed on lists.
list_ = ['Ajay', 'Vijay', 'Ramesh']
print (list_)
list_.append('Sujay') #Inserts Sujay at the end of the list
print (list_)
list_.insert(0, 'NewGuy') #Inserts NewGuy at 0 position
print (list_)
list_.extend(['Guy1', 'Guy2']) #Extends the list
print (list_)
print (list_.index('Guy1'))
list_.remove('Guy1') # Remove by Name
print (list_)
list_.pop(1) # Removes by location- Ajay is removed
print (list_)
list_.pop(-1) # Remove by location from behind
print (list_)
Try making your own list and apply the above-mentioned operations on your list!
Sorting Lists
We can also sort the list using the keyword “sort”. In lists that contain numbers, we can also find the maximum and minimum values in a string using the keywords “max” and “min”. Let’s get a clearer picture using an example:-
#Sorting
numberlist = [1, 5, 23, 1 ,54,2, 54,23, 54,76, 76,34,87]
numberlist.sort()
print(numberlist)
print (len(numberlist))
print (max(numberlist))
print (min(numberlist))
Tuples
A Tuple is a sequence of immutable Python objects. Tuples are sequences, just like Lists. The differences between tuples and lists are:
Tuples cannot be changed unlike lists
Tuples use parentheses, whereas lists use square brackets. Consider the example below:
Python = ('I', 'Understand' , 'Python')
So you must be wondering why use Tuples when we have Lists?
So the simple answer would be, Tuples are faster than lists. If you’re defining a constant set of values that you just want to iterate, then use Tuple instead of a List.
Now all Tuple operations are similar to Lists, but you cannot update, delete or add an element to a Tuple.
So put on your working gear and practice all operations on tuples.
Next in Python, let’s understand Strings.
Strings
Strings are amongst the most popular data types in Python. We can create them simply by enclosing characters in quotes (“ “). Python has very strong string processing capabilities.
Subsets of strings can be taken using the slice operator ( [ ] and [ : ] ) with indexes starting at 0 at the beginning of the string and working their way from -1 at the end.
The plus ( + ) sign is the string concatenation operator and the asterisk ( * ) is the repetition operator.
Strings in Python are immutable. Unlike other datasets such as lists, you cannot manipulate individual string values. To do so, you have to take subsets of strings and form a new string.
A string can be converted to a numerical type and vice versa (wherever applicable). Many times, raw data, although numeric, is coded in string format. This feature provides a clean way to make sure all of the data is in numeric form. For example:
a = '20'
anum = int(a) # Convert String '20' to Integer - Possible
print (anum + anum)
Now consider the following example:
strr = 'Hello World'
print (strr) # prints complete string
print (strr[0]) # prints first character of string
print (strr[2:6]) # prints characters starting from 3rd to 6th.'W' is excluded.
print (strr[2:]) # prints string starting from 3rd character
print (strr*2) # prints string two times
print (strr + "TEST") # prints concatenated string
Let us look at a few more operations that you can perform with strings:
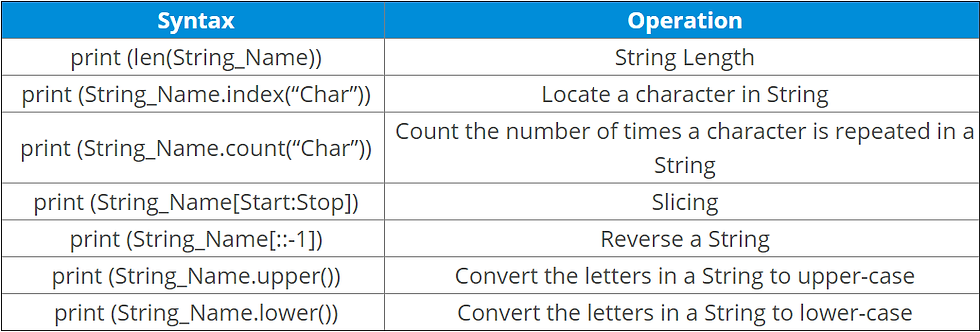
Let us see some more examples of operations on strings:
1.
string_ = "This is python"
print (string_.count('s'))
print (string_.split(' '))
print (string_.upper()) # Changes the string case to upper case
print (string_.lower()) # Changes the string case to lower case
print (string_.swapcase()) # Swaps the string cases
2.
school = 'PYTHON IS EASY'
print('A' in school) #The result would be 'true'
print('D' in school) #The result would be 'false'
You must practice these operations simultaneously so the concepts become clearer.
Next in Python Tutorial, it’s time to focus on the last Data type i.e. Dictionary
Dictionary
Dictionaries are one of the most important built-in data structure. They work like associative arrays and consist of key-value pairs. A dictionary key can be almost any Python type but are usually numbers or strings. Values, on the other hand, can be any arbitrary Python object.
Dictionaries are enclosed by curly braces ( { } ) and values can be assigned and accessed using square braces ( [] ).
Dict = {'Name' : 'Saurabh', 'Age' : 23}
For a clearer picture of how dictionaries work and operations on them let’s see some examples:
dict = {}
dict['one'] = "This is one"
dict[2] = "This is two"
tinydict = {'name': 'isb','code':6734, 'dept': 'sales'}
print (dict['one']) # Prints value for 'one' key
print (dict[2]) # Prints value for 2 key
print (tinydict) # Prints complete dictionary
print (tinydict.keys()) # Prints all the keys
print (tinydict.values()) # Prints all the values
Next up we’ll discuss the date and time format in python.
Date and time
Python has a built-in DateTime module for working with dates and times. One can create strings from date objects and vice versa.
Python offers very powerful and flexible DateTime capabilities that are capable of operating at a scale of time measured in nano-seconds. Let us look at some date time examples:
date1 = datetime(2014, 5, 16, 14, 45, 5) # Defining a date time variable
print(date1.day)
print(date1.month)
print(date1.year)
print(date1.second)
print(datetime.strptime('20140516134328','%Y%m%d%H%M%S')) #Parses a string representing a time according to a format
If an invalid format for strptime is entered there will be an error.
We can also find the time elapsed. Take a look at the following example:
datetime(2014, 5, 16)
date2 = datetime(2014, 5, 19)
datediff = date2 - date1
print(datediff)
Operators in Python
Operators are the constructs that can manipulate the values of the operands. Consider the expression 2 + 3 = 5, here 2 and 3 are operands and + is called operator.
Python supports the following types of Operators:
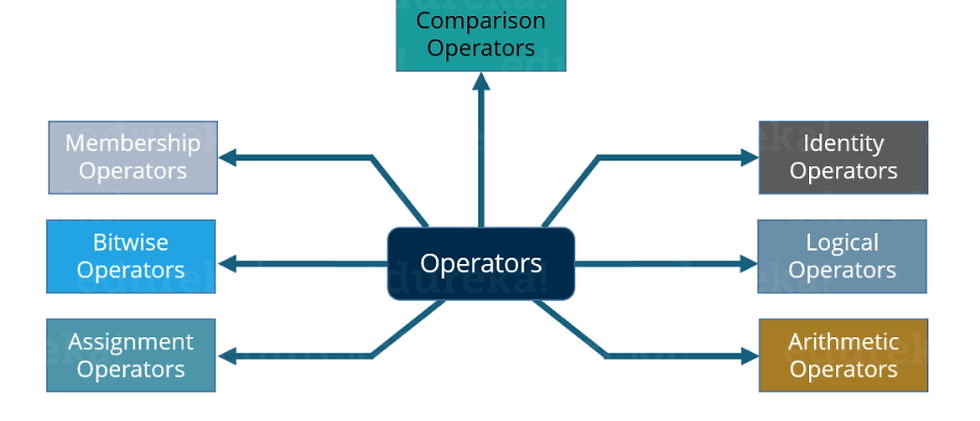
Let us focus now on each of these operators.
Arithmetic Operators:
These Operators are used to perform mathematical operations like addition, subtraction etc.
+ (plus) : Adds two objects.
- (minus): Gives the subtraction of one number from the other; if the first operand is absent it is assumed to be zero.
* (multiply): Gives the multiplication of the two numbers or returns the string repeated that many times.
** (power): Returns x to the power of y.
/ (divide) : Divide x by y.
// (floor division) : Returns the floor of the quotient.
% (modulo): Returns the remainder of the division.
a = 21
b = 10
c = 0
c = a + b
print ( c )
c = a - b
print ( c )
c = a * b
print ( c )
c = a / b
print ( c )
c = a % b
print ( c )
a = 2
b = 3
c = a**b
print ( c )
Output = 31, 11, 210, 2.1, 1, 8
Comparison Operators:
These operators compare the values on either side of them and decide the relation among them. All comparison operators return True or False. Note the capitalization of these names. Consider two variables x and y.
< (less than) : Returns whether x is less than y.
> (greater than) : Returns whether x is greater than y.
<= (less than or equal to) : Returns whether x is less than or equal to y.
>= (greater than or equal to) : Returns whether x is greater than or equal to y.
== (equal to) : Compares if the objects are equal.
!= (not equal to) : Compares if the objects are not equal.
a = 21
b = 10
c = 0
if ( a == b ):
print ("a is equal to b")
else:
print ("a is not equal to b")
if ( a != b ):
print ("a is not equal to b")
else:
print ("a is equal to b")
if ( a < b ): print ("a is less than b")
else: print ("a is not less than b")
if ( a > b ):
print ("a is greater than b")
else:
print ("a is not greater than b")
a = 5
b = 20
if ( a <= b ): print ("a is either less than or equal to b")
else: print ("a is neither less than nor equal to b")
if ( a => b ):
print ("a is either greater than or equal to b")
else:
print ("a is neither greater than nor equal to b")
Output :
a is not equal to b
a is not equal to b
a is not less than b
a is greater than b
a is either greater than or equal to b
a is either less than or equal to b
Now let us look at what assignment operators are.
Assignment Operators:
An Assignment Operator is an operator used to assign a new value to a variable.
=: Assigns values from right side operands to left side operand
+= (Add AND): It adds the right operand to the left operand and assigns the result to the left operand.
-= (Subtract AND): It subtracts the right operand from the left operand and assigns the result to the left operand.
*= (Multiply AND): It multiplies the right operand with the left operand and assigns the result to the left operand.
/= (Divide AND): It divides the left operand with the right operand and assigns the result to the left operand.
%= (Modulus AND): It takes modulus using two operands and assign the result to the left operand
**= (Exponent AND): Performs exponential (power) calculation on operators and assign value to the left operand.
a = 21
b = 10
c = 0
c = a + b
print ( c )
c += a
print ( c )
c *= a
print ( c )
c /= a
print ( c )
c = 2
c %= a
print ( c )
c **= a
print ( c )
Output : 31, 52, 1092, 52.0, 2097152, 99864
Bitwise Operators :
These operations directly manipulate bits. In all computers, numbers are represented with bits, a series of zeros and ones. In fact, pretty much everything in a computer is represented by bits.
Following are the Bitwise Operators supported by Python :
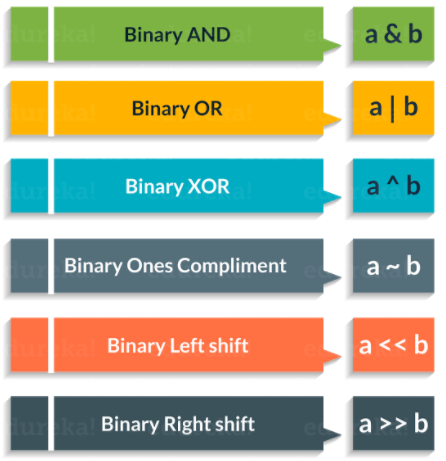
Consider the following example :
a = 58 # 111010
b = 13 # 1101
c = 0
c = a & b
print ( c ) # 8 = 1000
c = a | b
print ( c ) # 63 = 111111
c = a ^ b
print ( c ) # 55 = 110111
c = a >> 2
print ( c ) # 232 = 11101000
c = a << 2
print ( c ) # 14 = 1110
Output : 8, 63, 55, 232, 14
Next up, we’ll focus on Logical Operators.
Logical Operators
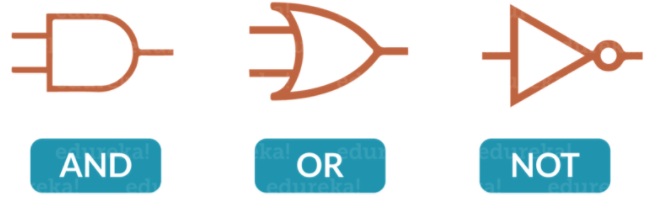
not (boolean NOT): If x is True, it returns False. If x is False, it returns True.
and (boolean AND): x and y return False if x is False, else it returns the evaluation of y.
or (boolean OR): If x is True, it returns True, else it returns the evaluation of y.
a = 6
b = 7
c = 42
print (1, a == 6)
print (2, a == 7)
print (3, a == 6 and b == 7)
print (4, a == 7 and b == 7)
print (5, not a == 7 and b == 7)
print (6, a == 7 or b == 7)
print (7, a == 7 or b == 6)
print (8, not (a == 7 and b == 6))
print (9, not a == 7 and b == 6)
Output :
True
False
True
False
True
True
False
True
False
Now in Python Tutorial, we’ll learn about Membership Operators.
Membership Operators:
These Operators are used to test whether a value or a variable is found in a sequence (Lists, Tuples, Sets, Strings, Dictionaries) or not. The following are the Membership Operators:
in: True if value/variable is found in the sequence.
not in: True if value/variable is not found in the sequence.
Consider the following example :
X = [1, 2, 3, 4]
A = 3
print(A in X)
print(A not in X)
Output :
True
False
Next up, it’s time we understand the last Operator i.e. Identity Operator.
Identity Operators :
These Operators are used to check if two values (or variables) are located on the same part of the memory. Two equal variables do not imply that they are identical.
Following are the Identity Operators in Python :
is: True if the operands are identical.
is not: True if the operands are not identical.
Consider the following example :
X1 = 'Welcome!'
X2 = 1234
Y1 = 'Welcome!'
Y2 = 1234
print(X1 is Y1)
print(X1 is not Y1)
print(X1 is not Y2)
print(X1 is X2)
Output :
True
False
True
False
Stay tuned for the next Python tutorial in which we will cover Conditional Statements, Loops, Functions, Exceptions and a lot more!
Till then keep practising and good luck!
Comments