Python Tutorial for Beginners - Part 2
- Darshita Kumar
- Jun 27, 2019
- 7 min read
Hello and welcome back to the second part of this tutorial!
If you haven't gotten a chance to go through the first part you can look into the suggested blogs under this blog!
In this tutorial, we'll cover the following topics:
Conditional Statements
Loops
Function
Modules
Python I/O Operation
Exceptions and Exception Handling
Let us get started then!
Conditional Statements
Conditional statements are used to execute a statement or a group of statements when some condition is true. There are namely three conditional statements - If, Elif, Else.
Let's understand how this actually works :
First, the control will check the 'If' condition. If it's true, then the control will execute the statements after the If condition.
When the 'If' condition is false, then the control will check the 'Elif' condition. If the Elif condition is true then the control will execute the statements after the Elif condition.
If the 'Elif' Condition is also false then the control will execute the Else statements.
Below is the syntax:
if condition1:
statements
elif condition2:
statements
else:
statements
Consider the following example :
X = 10
Y = 12
if X < Y: print('X is less than Y')
elif X > Y:
print('X is greater than Y')
else:
print('X and Y are equal')
Output = X is less than Y
Break statement
The break statement is used to break out of a loop statement i.e. stop the execution of a looping statement, even if the loop condition has not become False or the sequence of items has not been completely iterated over.
An important note is that if you break out of a for or while loop, any corresponding loop else block is not executed.
for i in range(1,10):
if i == 5:
break
print (i)
print('Done')
Output :
1
2
3
4
Done
Now it is time to understand loops.
Loops
In general, statements are executed sequentially. The first statement in a function is executed first, followed by the second, and so on
There may be a situation when you need to execute a block of code several times.
A loop statement allows us to execute a statement or group of statements multiple times.
The following diagram illustrates a loop statement:
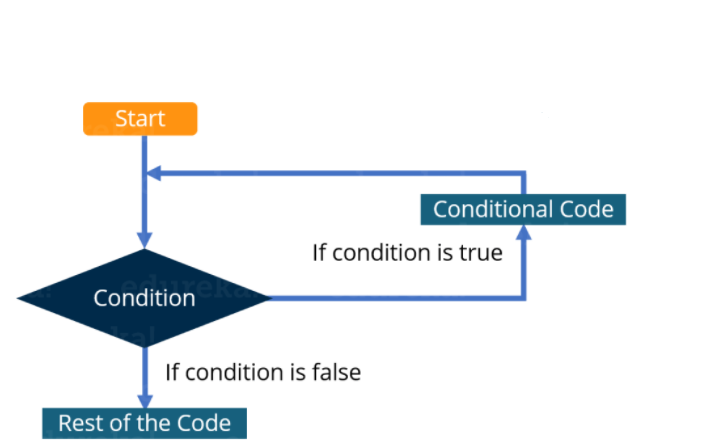
Let me explain to you the above diagram:
First, the control will check the condition. If it is true then the control will move inside the loop and execute the statements inside the loop.
Now, the control will again check the condition, if it is still true then again it will execute the statements inside the loop.
This process will keep on repeating until the condition becomes false. Once the condition becomes false the control will move out of the loop.
There are two types of loops:
Infinite: When condition will never become false.
Finite: At one point, the condition will become false and the control will move out of the loop.
There is one more way to categorize loops:
Pre-test: In this type of loops the condition is first checked and then only the control moves inside the loop.
Post-test: Here first the statements inside the loops are executed, and then the condition is checked.
Python does not support Post-test loops.
Loops in Python
In Python, there are three loops:
While
For
Nested
While Loop: Here, first the condition is checked and if it's true, control will move inside the loop and execute the statements inside the loop until the condition becomes false. We use this loop when we are not sure how many times we need to execute a group of statements or you can say that when we are unsure about the number of iterations.
Consider the example:
count = 0
while (count < 10):
print ( count )
count = count + 1
print ("Goodbye!")
Output :
0
1
2
3
4
5
6
7
8
9
Goodbye!
For Loop: Like the While loop, the For loop also allows a code block to be repeated a certain number of times.
The difference is, in For loop we know the number of iterations required unlike the While loop, where iterations depend on the condition.
You will get a better idea about the difference between the two by looking at the syntax:
for variable in Sequence:
statements
Notice here, we have specified the range, which means we know the number of times the code block will be executed.
Consider the example:
fruits = ['Banana', 'Apple', 'Grapes']
for index in range(len(fruits)):
print (fruits[index])
Output :
Banana
Apple
Grapes
Nested Loops: It basically means a loop inside a loop. It can be a For loop inside a While loop and vice-versa. Even a For loop can be inside a For loop or a While loop inside a While loop.
Consider the following example :
count = 1
for i in range(10):
print (str(i) * i)
for j in range(0, i):
count = count +1
Output :
1
22
333
4444
55555
666666
7777777
88888888
999999999
Now we would be focusing on functions.
Functions
Functions are a convenient way to divide your code into useful blocks, allowing us to order our code, make it more readable, reuse it and save some time. They are a reusable piece of software. They are blocks of statements that accepts some arguments, perform some functionality, and provides the output.
Functions are defined using the def keyword.
A function can also take arguments. Arguments are specified within parentheses in function definition separated by commas. It is also possible to assign default values to parameters to make the program flexible and not behave unexpectedly.
One of the most powerful features of functions is that it allows you to pass any number of arguments (*argv) and you do not have to worry about specifying the number when writing the function. This feature becomes extremely important when dealing with lists of input data where you do not know several data observations beforehand.
The scope of variables defined inside a function is local i.e. they cannot be used outside of a function.

Let us look at the following examples of functions :
# First example
def printMax(a, b):
if a > b:
print(a, 'is maximum')
elif a == b:
print(a, 'is equal to', b)
else:
print(b, 'is maximum')
printMax(3, 4)
OUTPUT : 4 is maximum
# Second example
def say(message, times = 1):
print(message * times)
say('Hello')
say('World ', 5)
OUTPUT : Hello
World World World World World
# Third example
def func(a, b=5, c=10):
print('a is', a, 'and b is', b, 'and c is', c)
func(3, 7)
func(25, c=24)
func(c=50, a=100)
OUTPUT : a is 3 and b is 7 and c is 10
a is 25 and b is 5 and c is 24
a is 100 and b is 5 and c is 50
# Fourth example
x = 50
def func(x):
print('x is', x)
x = 2
print('Changed local x to', x)
func(x)
print('x is still', x)
OUTPUT : x is 50
Changed local x to 2
x is still 50
# Fifth example
# *args will take any number of arguments as specified by the function
def print_two(*args):
arg1, arg2 =args
print("arg1 : %r , arg2: %r "%(arg1, arg2))
print_two("hey",2)
OUTPUT : arg1 : 'hey' , arg2: 2
Next up we have the concept of Modules.
Modules
Functions can be used in the same program but if you want to use function (s) in other programs, make use of modules. Modules can be imported into other programs and functions contained in those modules can be used.
The simplest way to create a module is to write a .py file with functions defined in that file.
Another way is to import using byte-compiled .pyc files.
Let's look at some examples of modules :
import math
x = -25
print(math.fabs(x))
print(math.factorial(abs(x)))
OUTPUT : 25.015511210043330985984000000
# Second example
import sys
sys.path.append(your_local_path)
from mymodule import *
print(sayhi())
OUTPUT : Hi, this is a function of mymodule.
None
# Third example
numb = input("Enter a non negative number: ") #Taking input from the user
num_factorial = factorial(int(numb))
OUTPUT : Enter a non negative number: 9362880
Python I/O Operation
How To Open A File Using Python?
Python has a built-in function open(), top open a file. This function returns a file object, also called a handle, as it is used to read or modify the file accordingly.
We can specify the mode while opening a file. In the mode, we specify whether we want to
read 'r'
write 'w' or
append 'a' to the file. We also specify if we want to open the file in text mode or binary mode.
The default is reading in text mode. In this mode, we get strings when reading from the file.
o = open("python.txt") # equivalent to 'r' or 'rt'
o = open ("python.txt",'w') # write in text mode
o = open ("img1.bmp",'rb' ) # read and write in binary mode
How To Close A File Using Python?
When we are done with operations to the file, we need to properly close the file.
Closing a file will free up the resources that were tied with the file and is done using the Python close() method.
o = open ("edureka.txt")
o.close()
The last concept that we'll be covering in this tutorial will be Exceptions and Exception Handling.
Exceptions
An exception is an event that interrupts the ordinary sequential processing of a program.
For example, what if you are going to read a file and the file does not exist? Or what if you accidentally deleted it when the program was running?
Similarly, what if your program had some invalid statements? Such situations are handled using exceptions.
Handling Exceptions
We can handle exceptions using the try..except statement. We basically put our usual statements within the try-block and put all our error handlers in the except-block.
Let's understand this by the following example :
def avg( numList ):
"""Raises TypeError or ZeroDivisionError exceptions."""
sum = 0
for v in numList:
sum = sum + v
return float(sum)/len(numList)
def avgReport(numList):
try:
m= avg(numList)
print ("Average = ", m)
except TypeError as ex:
print ("TypeError:", ex)
except ZeroDivisionError as ex:
print ("ZeroDivisionError:", ex)
list1 = [10,20,30,40] # If used this should runfine
list2 = [] #it would throw Zero Division Error
list3 = [10,20,30,'abc'] # If used this should throw TypeError
avgReport(list1)
print (avgReport(list2))
print (avgReport(list3))
Output :
Average = 25.0
ZeroDivisionError: float division by zero
None
TypeError: unsupported operand type(s) for +: 'int' and 'str'
None
I hope you have enjoyed reading this Python Tutorial.
We have covered all the basics of Python, so you can start practising now!
Good luck!
This post was written by Darshita.
Let me know your thoughts and thanks for reading!
Comments